Let’s look at some of the steps where we imagine that we read from a network card:
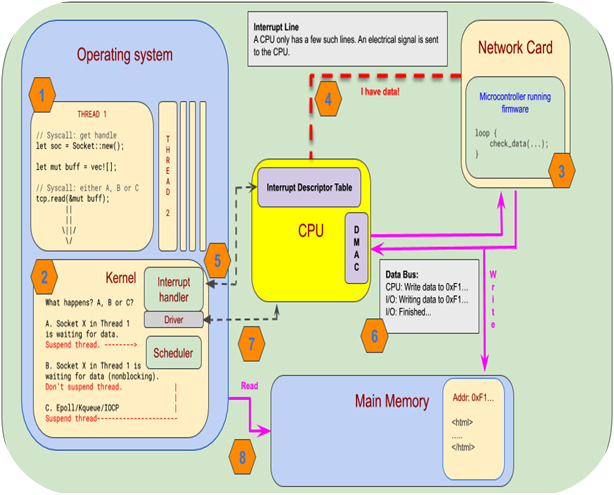
Remember that we’re simplifying a lot here. This is a rather complex operation but we’ll focus on the parts that are of most interest to us and skip a few steps along the way.
Step 1 – Our code
We register a socket. This happens by issuing a syscall to the OS. Depending on the OS, we either get a file descriptor (macOS/Linux) or a socket (Windows).
The next step is that we register our interest in Read events on that socket.
Step 2 – Registering events with the OS
This is handled in one of three ways:
- We tell the operating system that we’re interested in Read events but we want to wait for it to happen by yielding control over our thread to the OS. The OS then suspends our thread by storing the register state and switches to some other thread
From our perspective, this will be blocking our thread until we have data to read. - We tell the operating system that we’re interested in Read events but we just want a handle to a task that we can poll to check whether the event is ready or not.
The OS will not suspend our thread, so this will not block our code. - We tell the operating system that we are probably going to be interested in many events, but we want to subscribe to one event queue. When we poll this queue, it will block our thread until one or more events occur.
This will block our thread while we wait for events to occur.
Chapters 3 and 4 will go into detail about the third method, as it’s the most used method for modern async frameworks to handle concurrency.
Step 3 – The network card
We’re skipping some steps here, but I don’t think they’re vital to our understanding.
On the network card, there is a small microcontroller running specialized firmware. We can imagine that this microcontroller is polling in a busy loop, checking whether any data is incoming.
The exact way the network card handles its internals is a little different from what I suggest here, and will most likely vary from vendor to vendor. The important part is that there is a very simple but specialized CPU running on the network card doing work to check whether there are incoming events.
Once the firmware registers incoming data, it issues a hardware interrupt.